The ESP32 can support multiple network interfaces at once – be it Wi-Fi, ethernet or cellular modem. Using esp_netif APIs, you can search for valid interfaces and then bind sockets to a network interface or select a networking interface as default networking interface.
This note lists code snippets to search for network interfaces using the new esp_netif interface which is a part of latest Espressif ESP-IDF.
Using the esp_netif Network Interface Structure
esp_netif APIs take over the previous tcpip_adapter and allow an easier way to manage different network interfaces on the ESP32.
With the esp_netif API, you can manage DHCP, IP addresses, and other attributes of a physical network interface. This makes it easy for applications that have multiple network interfaces to switch network interfaces or select a default network interface for internet access.
The first step of binding a socket to a network interface is to identify the network interface, its hardware attributes and make sure that the interface actually exists. This firmware note has example code for scanning and searching valid netif interface objects on ESP32.
Searching Network Interfaces using esp_netif
The following code snippet creates a netif object pointer and assigns the first netif object to it. It keeps scanning for the next netif object or network interface and lists its unique name or descriptor.
When there is no valid network interface left, the esp_netif_next API returns NULL.
Note that passing NULL as argument to esp_netif_next() returns the first valid netif object in the current list of active network interfaces.
// Create an esp_netif pointer to store current interface
esp_netif_t *ifscan = esp_netif_next (NULL);
// Stores the unique interface descriptor, such as "PP1", etc
char ifdesc[7];
ifdesc[6] = 0; // Ensure null terminated string
while (ifscan != NULL)
{
esp_netif_get_netif_impl_name (ifscan, ifdesc);
ESP_LOGI (TAG, "IF NAME: %s", ifdesc);
// Get the next interface
ifscan = esp_netif_next (ifscan);
}
ESP_LOGI (TAG, "Done listing network interfaces");
Network Interface Scan Results, Example
When you run the netif interface scanner, you get a list like this. In this case, the only active interace is a 4G LTE modem registered as a PPPoS connection.
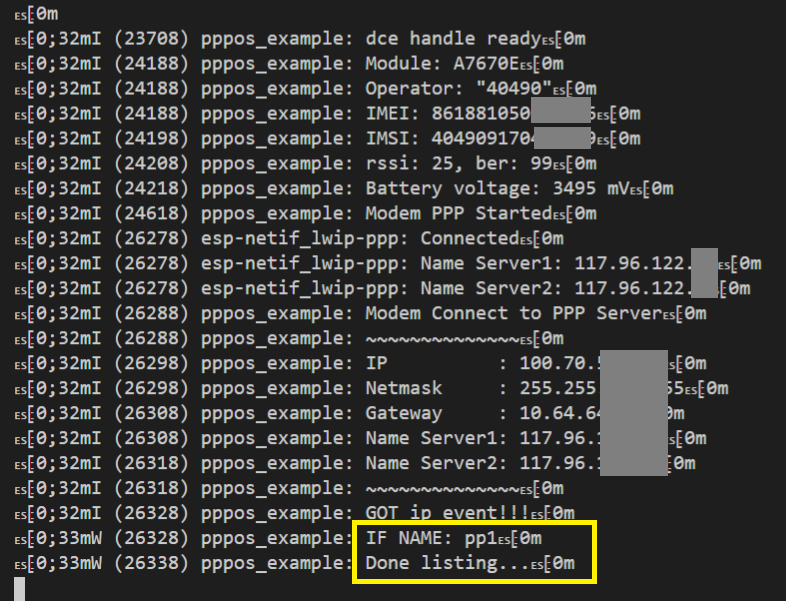
Feel free to ask away via the Quick Contact form in the sidebar, or leave a comment below.
Change Log
- Initial Release: 16 August 2021
References
- Reference 1: esp_netif API Official Documentation