The ESP32 from Espressif Systems is a very capable little SoC. What makes the ESP32 stand out is networking-friendly ESP-IDF that accompanies it. With ESP-IDF version 5.0, it has grown to provide Linux-like networking support to regular ESP32 applications. What would have required an OpenWRT based hardware setup in the past is now possible with ease using an ESP32. This article will walk you through the process of creating an ESP32 4G hotspot with easy to understand source code.
ESP32 4G Access Point Hardware
The ESP32 requires a cellular modem of some kind to access the internet. In this example, I have used one of our gateway devices that contains a SIM7600 modem from Simcom. The device is linked below, in case you are curious about its capabilities.
Note that the Wi-Fi access point is not the only option – you can also share the 4G cellular data link through the ethernet port of the ESP32. I will soon publish code and notes on that.
How the ESP32 4G Hotspot Works
ESP-IDF version 4.x started introducing some new experimental features that make it extremely easy to configure and use network interfaces flexibly. The key to ease of implementation of an access point is the following:
- netif API to manage networking interfaces
- PPP support to interface to the modem in data mode
- NAT support to allow effortless translation of packets across networks
Please stick to ESP-IDF 5.0.1. Older versions of ESP-IDF may cause a random crash in esp_netif_internal_dhcpc_cb() callback. It appears to be a bug.
ESP-IDF Example Code and Notes
The 4G hotspot program is easy to follow for a beginner. The code is based on the ap_to_pppos example of Espressif’s esp_modem component.
However, just to make things reusable and easily readable, I have reorganized the code to make a lot of the modem configuration and setup code re-usable as an ESP-IDF component. This cleans up user code in new applications and makes it easy to re-use the Gateway driver code.
If you would like to take a look at the example for ESP32 4G hotspot, here is the GitHub link.
The code execution flow is described below. You can easily modify features and behavior to make variants of the 4G hotspot – for example, you can also make an ethernet to 4G router with the ESP32.
Let’s walk through the app_main() of the example code.
Initialize the ESP32 core
// Initialize NVS
esp_err_t ret = nvs_flash_init();
if (ret == ESP_ERR_NVS_NO_FREE_PAGES || ret == ESP_ERR_NVS_NEW_VERSION_FOUND) {
ESP_ERROR_CHECK(nvs_flash_erase());
ret = nvs_flash_init();
}
ESP_ERROR_CHECK(ret);
/* Init and register system/core components */
ESP_ERROR_CHECK(esp_netif_init());
ESP_ERROR_CHECK(esp_event_loop_create_default());
The first and obvious step is to set up the ESP32 which is connected to the 4G modem.
NVS flash needs to be initialized for Wi-Fi parameter storage.
esp_netif needs to be initialized at the very beginning to allow networking over Wi-Fi and PPPoS.
A default event loop handler is initialized to catch up with any network events.
Initialize the SIM7600 modem
pcbartists_modem_power_up_por ();
pcbartists_modem_setup ();
The pcbartists_modem_power_up_por() defined in gw_modem.c performs a complete power cycle of the modem to restore it to its default state.
This is important to ensure that the modem is in a “known” state and responds well to commands.
The ESP32 resetting itself because of a fault or during testing might leave the SIM7600 in an unpredictable state. Therefore, the reset is highly recommended even though it takes about 30 seconds.
In low power applications, this can be avoided to save power as long as the ESP32 maks sure the modem is put to sleep and woken up properly.
pcbartists_modem_setup() manages a whole lot of DCE and DTE initialization code so you don’t have to. It initializes and manages modem event handling as well and ensures that the modem AT core is initialized. Finally, it sets the modem up for 4G connectivity and puts the modem UART port into PPP data mode instead of the AT command mode.
Wait for 4G data connection
// Wait for IP address
ESP_LOGI(TAG, "Waiting for IP address");
xEventGroupWaitBits (pcbartists_modem_eventgroup(), MODEM_CONNECT_BIT, pdFALSE, pdFALSE, portMAX_DELAY);
// Modem is now connected to the 4G network
Code now has to wait for the modem event group to register an active internet connection. This can be checked by reading the MODEM_CONNECT_BIT, which is set when an IP address is acquired by the modem.
portMAX_DELAY sets the wait timeout duration to forever. In a power sensitive application, this timeout can be set to 30 seconds. If the MODEM_CONNECT_BIT is not set within that time frame, the modem can be put into a low power state to retry connection some other time.
Configure netif and DNS
// Proceed to set up WiFi AP and NAT
esp_netif_t *ap_netif = esp_netif_create_default_wifi_ap();
assert(ap_netif);
esp_netif_dns_info_t dns;
esp_netif_t *modem_netif = pcbartists_get_modem_netif();
assert (modem_netif);
// Fetch DNS IP from modem netif
ESP_ERROR_CHECK(esp_netif_get_dns_info(modem_netif, ESP_NETIF_DNS_MAIN, &dns));
// Assign DNS for the AP
set_dhcps_dns(ap_netif, dns.ip.u_addr.ip4.addr);
The ESP32 AP is attached as a network interface and is pointed to by ap_netif.
The modem has already been added to netif management when pcbartists_modem_setup() was called. It is simply read back into modem_netif.
esp_netif_get_dns_info() extracts DNS server information from modem_netif. This DNS information is then assigned to the AP netif so end devices connected to the AP can access the same DNS server.
Start the Wi-Fi hotspot and NAT
// WiFi AP init
wifi_init_softap();
ip_napt_enable(_g_esp_netif_soft_ap_ip.ip.addr, 1);
ESP_LOGW (TAG, "Hotspot should now be functional...");
Finally, the Wi-Fi access point or hotspot is created so that other Wi-Fi devices can discover the ESP32 Wi-Fi network and connect to it. Because this is a hotspot, you do have control over the channel to be used, network name and authentication method.
ip_napt_enable() enables NAT or NAPT (network port/address translation) on the ESP32 hotspot.
The ESP32 hotspot is ready! You can connect your phone to the hotspot and browse the internet to make sure the hotspot is functional.
Debug Log for Reference
If everything is configured properly and all goes well, you should see debug logs like this.
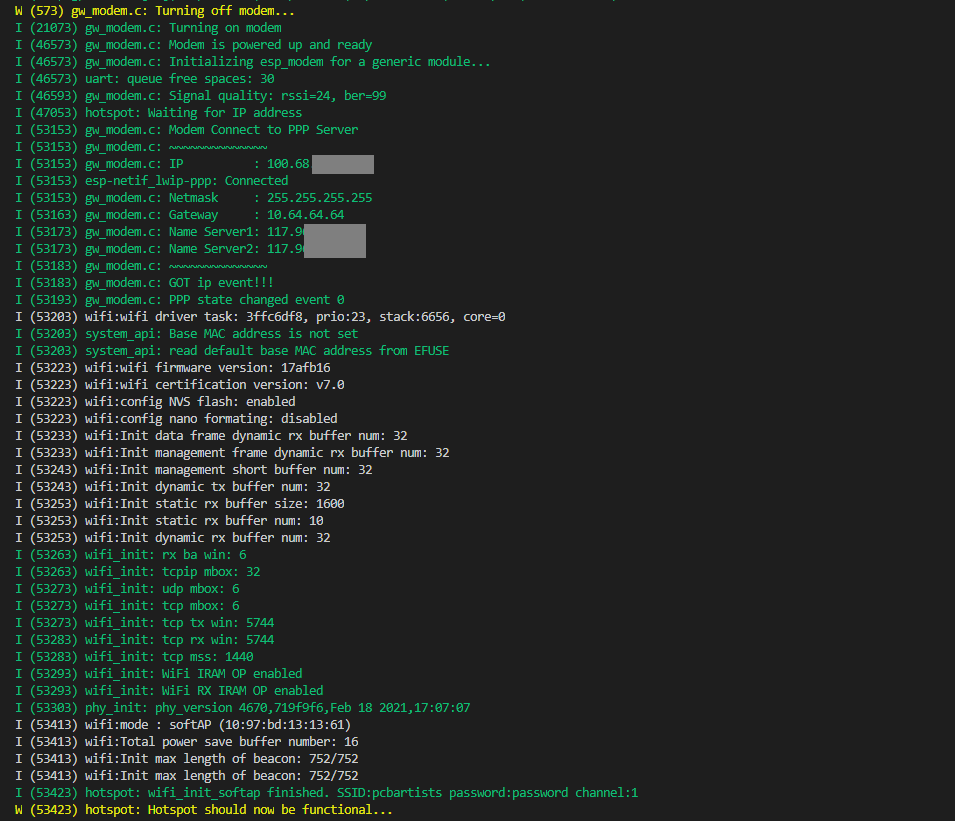
Have Something to Say?
Feel free to ask away via the Live Chat, drop us a message using the Quick Contact form in the sidebar, or leave a comment below.
Change Log
- 25 February 2023
– Initial release